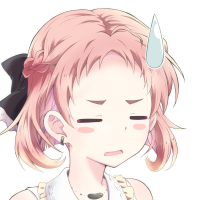
Python
is one of the easiest programming languages to learn.
Same as other languages, Python also has loop procedure.
Loop continues until loop count or element reaches to end.
But if we achieve the purpose, we would like to stop loop.
And we may not want to process some data.
In that case, what should we do ?
So today I will introduce ”How to get out of loop or skip loop in Python”.
Author
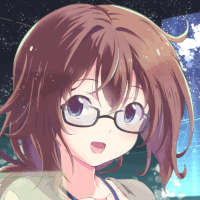
Advantage to read
You can understand "How to get out of loop or skip loop in Python".
for loop in Python
First, write "for loop" in Python.
# normal loop for i in range(0,3): print("i:{}".format(i)) for j in range(0, 2): print(" j:{}".format(j)) # i:0 # j:0 # j:1 # i:1 # j:0 # j:1 # i:2 # j:0 # j:1
This case I prepared double loop.
Accoording to document, we can write other type of loop.
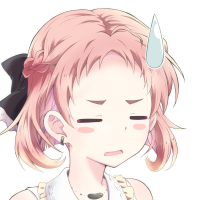
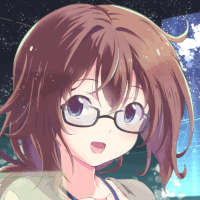
How to get out of loop in Python
In order to get out of loop in Python, use break
.
You can use break
like below.
# break loop1 for i in range(0,3): print("i:{}".format(i)) if i == 1: print("break") break for j in range(0, 2): print(" j:{}".format(j)) # i:0 # j:0 # j:1 # i:1 # break
In previous code, we could see the step i=2
.
But this case, break
is used in step i=1
.
So the loop was stopped.
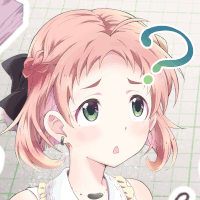
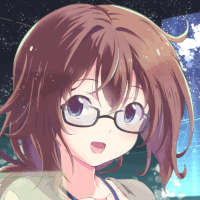
What will happen if we break
in second loop.
If we do, we can see the result below.
# break loop2 for i in range(0,3): print("i:{}".format(i)) for j in range(0, 2): print(" j:{}".format(j)) if j == 0: print("break") break # i:0 # j:0 # break # i:1 # j:0 # break # i:2 # j:0 # break
I put break
in loop j.
So the loopwas stopped in step j=0
.
And loop i seems continued.
So it means that break
stops nearest loop.
How to skip loop iteration in Python
Then how can we skip loop iteration in Python ?
You can use continue
to do it.
# skip loop for i in range(0,3): print("i:{}".format(i)) if i == 1: print("continue") continue for j in range(0, 2): print(" j:{}".format(j)) # i:0 # j:0 # j:1 # i:1 # continue # i:2 # j:0 # j:1
With using continue
, we skipped loop iteration of i=1
.
And the loop continued and executed loop iteration of i=2
.
Like this, we can use continue
to skip loop iteration that matches specific condition.
Finally
Today I introduced ”How to get out of loop or skip loop in Python”.
In order to control loop, we can use these methods.
Loop control tools
There is document about loop control.
If you want to know more, it is good to read it.